Gulp is a flexible and powerful tool to set up task execution that helps to automate development routines. The following is an explanation of how to automate CSS compilation and processing from Sass files using Gulp for a PHP project in PhpStorm IDE.
I would like to use Sass in a project that I develop in PhpStorm. The project already uses a third-party CSS framework that I would like to keep intact. My customizations will include several style overrides and a few custom CSS style definitions. Because of this, I would like to keep my source and distribution files separate.
Install Gulp
I would like to use Gulp to compile and minify my stylesheets. This will require Node.js npm utility. For more information, read this guide on how to get npm.
Within my project, I first need to initialize a new Node project, and install Gulp and Gulp CLI. To type the commands, I open the PhpStorm terminal with Alt + F12
:
npm init -y
npm install --save-dev gulp
npm install --save-dev gulp-cli
For my goals, I will need several Gulp plugins:
gulp-sass
– to compile Sass source files into CSSdel
– to clean up the distribution directorygulp-clean-css
– to minify CSSgulp-rename
– to rename minified CSS filesgulp-sourcemaps
– to add source maps to the minified files
I install these plugins as development dependencies:
npm i --save-dev gulp-sass
npm i --save-dev del
npm i --save-dev gulp-clean-css
npm i --save-dev gulp-rename
npm i --save-dev gulp-sourcemaps
Once that is done, I can see them in my projects settings (Ctrl + Alt + S
)
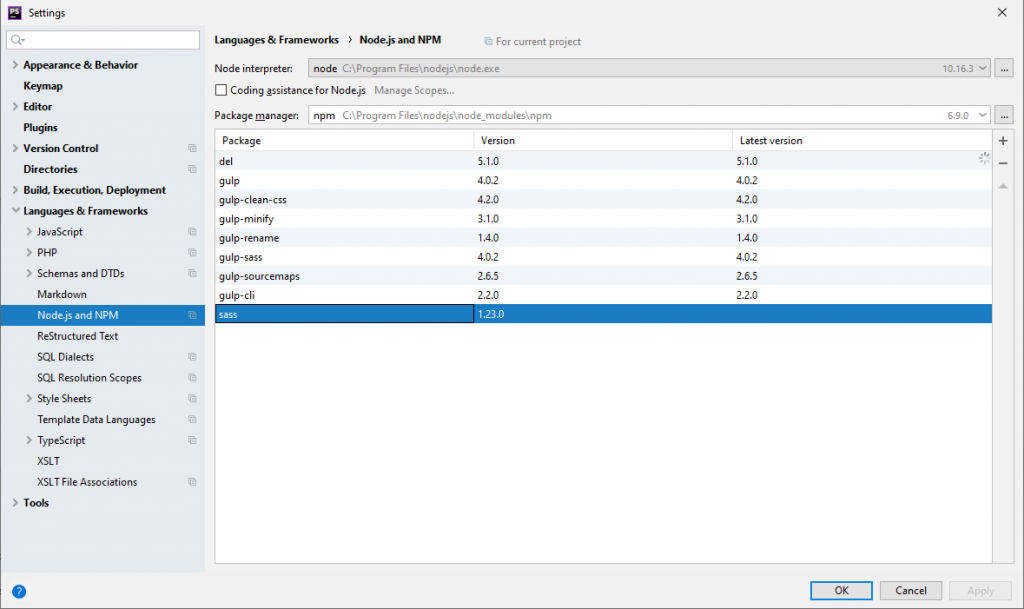
Setup Sass processing in the gulpfile.js
Next, I create the gulpfile.js
in my project root and add the following constants first:
const gulp = require('gulp');
const sass = require('gulp-sass');
const del = require('del');
const cleanCSS = require('gulp-clean-css');
const sourcemaps = require('gulp-sourcemaps');
const rename = require('gulp-rename');
// Style working directory
const sourceDir = 'wp-content/themes/my_theme/sass/';
// Style dist directory
const distDir = 'wp-content/themes/my_theme/css/my_styles/';
The first constants represent functions I import from Gulp plugins for build tasks. The last two constants, sourceDir
and distDir
, are folders (in this project, within a WordPress theme) where I will put my source Sass files and where the distribution files will be placed. This folder structure looks like this:
wp-content
themes
my_theme
sass # source Sass files
css
my_styles # distribution folder for the processed CSS
Next, I add the first task to this file. This task will compile Sass files to CSS:
gulp.task('styles', () => {
return gulp.src(sourceDir + '**/*.scss')
.pipe(sass().on('error', sass.logError))
.pipe(gulp.dest(distDir));
});
The next task will clean up the distribution folder. Previous versions of compiled files must be removed before newly compiled ones can be copied there:
gulp.task('clean', () => {
return del([
distDir + '*.css',
]);
});
The following task will minify the freshly compiled CSS files and save them with .min.css
extension. Also, it writes source maps:
gulp.task('minify-css', () => {
return gulp.src(distDir + '*.css')
.pipe(sourcemaps.init())
.pipe(cleanCSS({debug: true}, (details) => {
console.log(`${details.name}: ${details.stats.originalSize}`);
console.log(`${details.name}: ${details.stats.minifiedSize}`);
}))
.pipe(sourcemaps.write())
.pipe(rename({suffix: '.min'}))
.pipe(gulp.dest(distDir));
});
This task will watch over any changes in my source Sass files and execute a series of task to compile, and minify CSS files:
gulp.task('watch', () => {
gulp.watch(sourceDir + '*.scss', (done) => {
gulp.series(['clean', 'styles', 'minify-css'])(done);
});
});
Finally, I will add a default task that is executed every time I run gulp
command on my project without specifying a task:
gulp.task('default', gulp.series(['clean', 'styles', 'minify-css']));
Running Gulp tasks in PhpStorm
To open the Gulp task window, I need to right-click my gulpfile.js
and select the Show Gulp Tasks menu. This will open the following window:
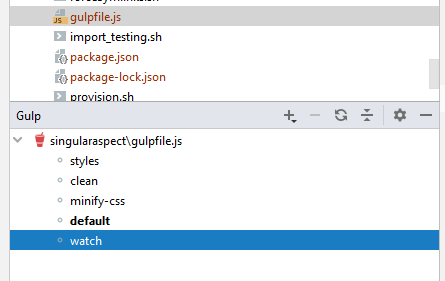
If I choose to run the watch
task, it will continue executing in the background doing its thing every time I save a source Sass file:
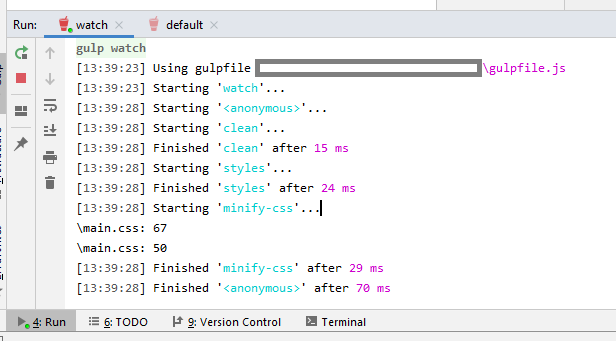
See the official JetBrains documentation on additional information on the use of Gulp in PHPStorm.
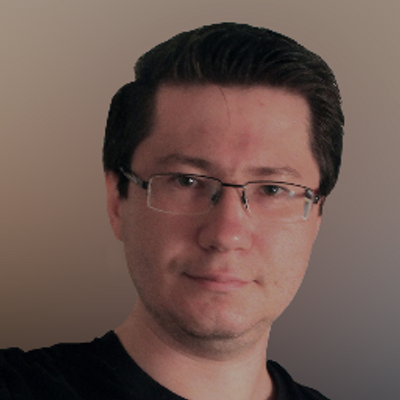
0 Comments